728x90
Dog or Cat
개와 고양이 사진을 주었을때 제대로 구분해 낼 수 있는 CNN모델을 구축해보도록 하자.
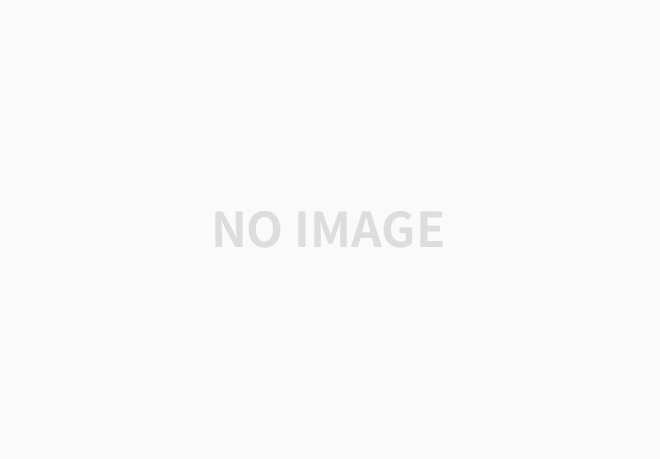
Importing the libraries
import tensorflow as tf
from keras.preprocessing.image import ImageDataGenerator
tf.__version__
Part 1 - Data Preprocessing
Preprocessing the Training set
train_datagen = ImageDataGenerator(rescale = 1./255,
shear_range = 0.2,
zoom_range = 0.2,
horizontal_flip = True)
training_set = train_datagen.flow_from_directory('dataset/training_set',
target_size = (64, 64),
batch_size = 32,
class_mode = 'binary')
Preprocessing the Test set
test_datagen = ImageDataGenerator(rescale = 1./255)
test_set = test_datagen.flow_from_directory('dataset/test_set',
target_size = (64, 64),
batch_size = 32,
class_mode = 'binary')
Part 2 - Building the CNN
Initialising the CNN
cnn = tf.keras.models.Sequential()
Step 1 - Convolution
cnn.add(tf.keras.layers.Conv2D(filters=32, kernel_size=3, activation='relu', input_shape=[64, 64, 3]))
Step 2 - Pooling
cnn.add(tf.keras.layers.MaxPool2D(pool_size=2, strides=2))
Adding a second convolutional layer
cnn.add(tf.keras.layers.Conv2D(filters=32, kernel_size=3, activation='relu'))
cnn.add(tf.keras.layers.MaxPool2D(pool_size=2, strides=2))
Step 3 - Flattening
cnn.add(tf.keras.layers.Flatten())
Step 4 - Fully connected layer
cnn.add(tf.keras.layers.Dense(units=128, activation='relu'))
Step 5 - Output Layer
cnn.add(tf.keras.layers.Dense(units=1, activation='sigmoid'))
Part 3 - Training the CNN
Compiling the CNN
cnn.compile(optimizer = 'adam', loss = 'binary_crossentropy', metrics = ['accuracy'])
Training the CNN on the Training set and evaluating it on the Test set
cnn.fit(x = training_set, validation_data = test_set, epochs = 25)
Part 4 - Making a single prediction
import numpy as np
from keras.preprocessing import image
test_image = image.load_img('dataset/single_prediction/cat_or_dog_1.jpg', target_size = (64, 64))
test_image = image.img_to_array(test_image)
test_image = np.expand_dims(test_image, axis = 0)
result = cnn.predict(test_image)
training_set.class_indices
if result[0][0] == 1:
prediction = 'dog'
else:
prediction = 'cat'
print(prediction)
개, 고양이의 사진을 넣었을때 정확하게 dog와 cat을 출력하는 것을 볼 수 있었다.
728x90
'Robotics & AI > DeepLearning' 카테고리의 다른 글
Udemy - 딥러닝의 모든 것(SOM)-(2) (1) | 2024.01.03 |
---|---|
Udemy - 딥러닝의 모든 것(SOM)-(1) (0) | 2023.09.12 |
GAN (0) | 2023.07.26 |
CNN for NLP (0) | 2023.07.22 |
딥러닝 직접 구현하기 - (신경망 학습) (0) | 2023.07.06 |